
April 12 is my 6 month anniversary at Microsoft, and also
happens to be the date we've launched .NET 4 and Visual Studio
2010. Congratulations to the product teams who made this release
happen!
.NET 4 has had a ton of improvements, and Visual Studio 2010
with the new WPF interface and MEF-based plug-in model is an
amazing piece of software. Having watched it grow from the
different loosely-related IDEs (Visual Interdev anyone?) to the
cohesive application is now has been a great process, and is
something that makes me proud to work at Microsoft.
Enough gushing, let's take a look at one of the areas in .NET of
particular interest to me: WPF.
Download .NET 4 and tools
or
Once you have the tools installed, you'll be ready to get
started. Check out the resources below for some places to visit
before you embark.
WPF 4 Resources
What's New in WPF 4 - feature review
.NET 4 is the best .NET release to date. I'm really proud of
what the teams accomplished here, from the new features in WPF to
integrating MEF, to the performance improvements, to the newer,
smaller client runtime. Awesome stuff.
Throughout the rest of this post, I'm going to walk through the
features that I think are important for WPF developers.
Visual Studio
2010
WPF Tracing Support
WPF Designer
Text
New Text Rendering Stack
ClearTypeHint
Selection and Caret Customization
Custom Dictionaries API
Graphics
Layout Rounding
Cached Composition
Pixel Shader 3
New Pixel Shader APIs
Easing Functions
Removal of legacy bitmap effects
Controls
DataGrid
Calendar
DatePicker |
Binding
Binding Commands on InputBinding
Bind to Dynamic Objects
Bindable Text Run
Styling and
Templating
Visual State Manager
Windows 7
Multi-Touch and Manipulation
Integration with the Shell and Taskbar
General
Client Profile
Managed Extensibility Framework
Parallel Computing
XBAP Script Access
|
Visual Studio 2010 has had a complete overhaul on the UI side.
However, did you know we threw in a few WPF goodies just to round
things out?
WPF Tracing Support
This is my favorite. Tracing in WPF used to involve messing
around with registry keys and config files. It wasn't fun. Now, all
you need to do is enable tracing in Visual Studio 2010
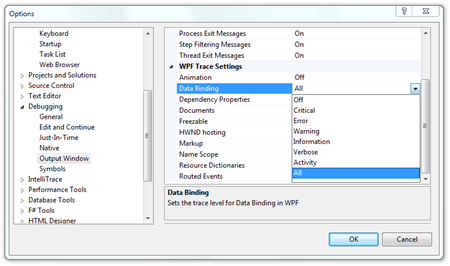
The new Visual Studio WPF/Silverlight Design Surface -
Cider
The new designer in Visual Studio is better than ever. I'm a
xaml editor kind of guy, but I can't help but be impressed with the
new designer's capabilities.
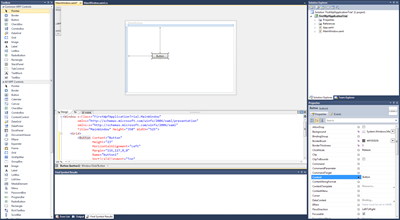
Not only do you get all the usual control placement and
alignment tools, but binding elements, or extracting them as
resources is only a context menu away.
For many, the binding syntax is the first hurdle to
understanding WPF and Silverlight. Below are three screenshots
showing setting the data context of the window to the current
employee, then binding a TextBox to a property of that object. No
curly braces required :)
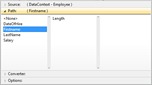
For more information on what's new in Visual Studio 2010, check out the
MSDN page here.
In previous versions of WPF, we provided a decent, but not
perfect, text rendering implementation. We concentrated more on
making sure subpixel layout of text worked correct, scaled
correctly, animated correctly and otherwise behaved well in your
applications. At the same time, however, we've heard you loud and
clear on how you want text in WPF to be as crisp and clean as
GDI/DirectWrite rendered text.
New Text Rendering Stack - GDI Compatible Text
The text stack in WPF 4 was rewritten from the ground up to
offer you the option to display text that is indistinguishable from
native applications in Windows. Here's a screenshot of
GDI-compatible WPF text.
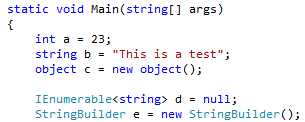
ClearTypeHint
The new Clear Type Hint API provides more control over when
ClearType is used. Essentially, you're helping the text and
rendering stacks make more educated decisions about where and when
to apply ClearType font smoothing.
Selection and Caret Customization
WPF 4 adds the ability to change the brushes for the caret and
selection text inside TextBox and similar controls.
<TextBox Text="Custom Selection"
Width="450"
Height="80"
FontSize="50"
Margin="10">
<TextBox.SelectionBrush>
<LinearGradientBrush StartPoint="0,0"
EndPoint="1,0">
<GradientStop Offset="0"
Color="Red" />
<GradientStop Offset="0.5"
Color="Yellow" />
<GradientStop Offset="1.0"
Color="Blue" />
</LinearGradientBrush>
</TextBox.SelectionBrush>
</TextBox>
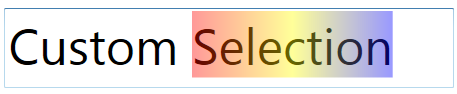
Custom Dictionaries API
WPF has had a spell-checker enabled for some time. However, it
has always used the OS spell checker. WPF 4 now includes the
ability to provide additional custom dictionaries to use for spell
checking.
Where would WPF be without its great graphics stack? WPF 4 adds
some nice enhancements in the quality, performance and capability
areas.
Layout Rounding
WPF and Silverlight are both optimized for subpixel layout. That
allows you to align elements on partial pixels. However, that can
often lead to single-pixel lines that are too thick, and "fuzzy
looking". Layout rounding lets you take a rectangle that (zoomed
in) looks like this:
![image_thumb[3][1]](/media/38866/WindowsLiveWriter_WhatsNewinWPF4Release_D6D6_image_thumb[3][1]_771ce543-ad31-444a-ac63-cbbdd26bd17c.png)
And make sure it looks like this:
![image_thumb[5][1]](/media/38871/WindowsLiveWriter_WhatsNewinWPF4Release_D6D6_image_thumb[5][1]_3885b188-a204-4c7a-9202-620c680ed077.png)
Why use this instead of pixel snapping? Pixel snapping didn't
work in many situations due to its render-time calculations. Layout
rounding is more predictable and, as a bonus, is
Silverlight-compatible.
Cached Composition
In this release, we introduced the concept of
hardware-accelerated cached composition. This can be a huge win for
render-heavy layouts that are cache-friendly, particularly layouts
with lots of vector elements.
Cached Composition is now supported directly in the framework
via the UIElement.CacheMode property and the BitmapCacheBrush. The
BitmapCacheBrush is essentially a a visual brush which pulls from
the cache.
One interesting aspect of the bitmap cache is the elements
remain interactive even while cached. Impressive, most impressive
:)
Pixel Shader 3 Support
WPF now supports Shader Model 3 pixel shaders (ps3.0). That
means you can now create even more interesting and complicated
effects. the PS3 model enables more instructions, more registers,
more texture indirections and better flow control. One caveat,
however, is Pixel Shader 3 has no software fallback.
New Pixel Shader APIs
To check the capabilities of the end-user's machine, you can use
the RenderCapability class with the existing
IsPixelShaderVersionSupported function, as well as two new
functions:
bool supported = RenderCapability.IsPixelShaderVersionSupported(3, 0);
bool software = RenderCapability.IsPixelShaderVersionSupportedInSoftware(3, 0);
int slots = RenderCapability.MaxPixelShaderInstructionSlots(3, 0);
The class has tons of other useful properties and methods as
well. It's a good practice to check the capabilities of the machine
and then cleanly downgrade functionality if the required support is
not present.
Removal of legacy Bitmap Effects
The old legacy bitmap effects (bevel, drop shadow, blur etc.)
are still present, but there's zero implementation. The effects
that have shader versions (blur, drop shadow) get forwarded to the
shader implementation. The other effects (bevel, for example)
simply do nothing. Your code will compile, but the effects
themselves are no-ops. In .NET 3.5sp1, they were marked as
deprecated, so this is not surprising.
Bitmap effects are software-only, and couldn't be hardware
accelerated. One of the biggest performance issues with old WPF
applications was use/overuse of bitmap effects. Pixel shader-based
effects are much more performant. I'm glad to see we aren't afraid
to cull when we come up with something better.
The replacement effects are just as easy to use as the old
effects, and will even render on the design surface.
// legacy
<Ellipse Width="250"
Height="250" Fill="Red">
<Ellipse.BitmapEffect>
<DropShadowBitmapEffect />
</Ellipse.BitmapEffect>
</Ellipse>
// current
<Ellipse Width="250"
Height="250" Fill="Red">
<Ellipse.Effect>
<DropShadowEffect />
</Ellipse.Effect>
</Ellipse>
Easing Functions
Easing functions are a great way to add bounce and pop to your
animation. There is a prebuilt set of functions as well as the
capability to create your own easing functions. Easing functions
simply alter the value over time either on the in or outgoing
event. The xaml below shows an easing function in use.
<DoubleAnimationUsingKeyFrames Storyboard.TargetName="Transform"
Storyboard.TargetProperty="ScaleX">
<EasingDoubleKeyFrame KeyTime="0:0:0"
Value="0.0" />
<EasingDoubleKeyFrame KeyTime="0:0:3"
Value="2.0">
<EasingDoubleKeyFrame.EasingFunction>
<ElasticEase EasingMode="EaseOut" />
</EasingDoubleKeyFrame.EasingFunction>
</EasingDoubleKeyFrame>
</DoubleAnimationUsingKeyFrames>
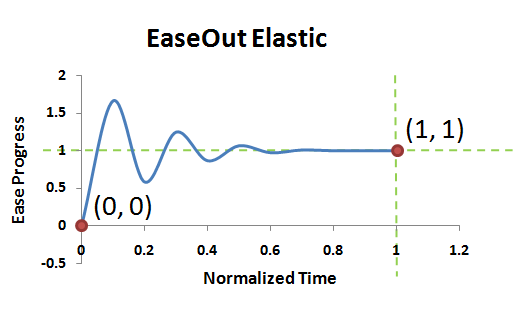
I wrote a blog post some time ago explaining how to use easing
functions in WPF 4 and Silverlight. Also, there is an accompanying
video and another blog/video pair explaining how to create your own
custom easing functions.
DataGrid, Calendar, DatePicker
I'm happy to see all of these controls make it into the
framework proper. The DataGrid, in particular, is an
accomplishment. If you've ever tried to write a grid control of
your own, or you looked at the DataGrid source on codeplex, you
know that creating a datagrid type control is a serious amount of
engineering.
This is also another case of releasing things early and often,
via codeplex (with source), and then when they mature, including
them into the framework itself. I'm pretty happy with this
model.
Binding Commands on InputBinding
You can now bind commands to input bindings. For example, you
can associate a command with a KeyBinding on a treeview or menu.
This better supports patterns like MVVM/ViewModel where you want to
associate all your UI to VM actions via ICommand set via
binding.
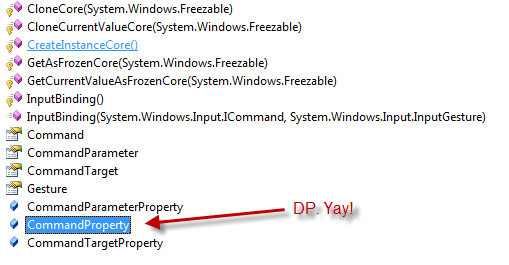
Yes, that means you can now write code like this:
<TreeView.InputBindings>
<KeyBinding Key="Delete" Command="{Binding RemoveItemCommand}"/>
</TreeView.InputBindings>
Bind to Dynamic Objects
If you can access a property in code, you can now bind to
it.
But now we get more than just anonymous types: we can bind to
dynamic types, even those resolved at runtime. This means you can
even data bind to those IDispatch COM objects you've been using to
automate Office, and use binding with the DLR.
Bindable Run
Speaking of binding, you can now bind to runs of text inside a
TextBlock or a FlowDocument. This allows you to compose more
complex text, that still flows correctly, without having to resort
to custom code.
<TextBlock>
<Run Text="Hello " />
<Run Text="{Binding Salutation}" />
<Run Text=" " />
<Run Text="{Binding FirstName}" />
<Run Text=" " />
<Run Text="{Binding LastName}" />
<Run Text="," />
</TextBlock>
Binding is essential to WPF and Silverlight development. The
more that's bindable, the happier I am.
Visual State Manager
This is one instance where Silverlight (along with Expression)
introduced something incredibly awesome, and WPF has been able to
pick it up and include it in the full framework. VSM has been
available to WPF developers since .NET 3.5sp1, but it was in a
toolkit library at the time. The syntax hasn't changed, though, so
what you learned with 3.5sp1 and with Silverlight all continues to
apply.
Multi-Touch and Manipulation
Windows 7 is the first Microsoft desktop OS to bring multi-touch
capabilities as part of the core. WPF 4 does a great job in
surfacing those multi-touch and manipulation capabilities for use
in our own code. While you get baked-in touch capabilities in
controls deriving from UIElement, and automatic promotion to mouse
events, the main events you'll want to use are the
ManipulationStarting, ManipulationDelta, and
ManipulationInertiaStarting . Those let you create a truly custom
touch experience with your own gestures, inertia and physics.
Integration with the Windows 7 Shell and Taskbar

WPF 4 provides a full suite of integration points with the
Windows 7 shell and taskbar. We get OS-specific common dialogs
(Windows 7 and Vista) including the ability to customize them using
FileDialogCustomPlace, as well as the ability to overlay status and
icons on the taskbar, control the jump list, add icons to the
thumbnail window and more. If you really want to light up on
Windows, WPF makes it easy for you, especially with the new
TaskbarItemInfo class.
<Window.TaskbarItemInfo>
<TaskbarItemInfo>
<TaskbarItemInfo.ThumbButtonInfos>
<ThumbButtonInfo ImageSource="{StaticResource Button1Image}"
Click="Button1_Click"
Description="Button 1" />
<ThumbButtonInfo ImageSource="{StaticResource Button2Image}"
Click="Button2_Click"
Description="Button 2"
IsEnabled="False"
IsBackgroundVisible="True"
Visibility="Visible"/>
<ThumbButtonInfo ImageSource="{StaticResource Button3Image}"
Click="Button3_Click"
Description="Button 3" />
</TaskbarItemInfo.ThumbButtonInfos>
</TaskbarItemInfo>
</Window.TaskbarItemInfo>

There are a number of good videos and blog posts on these
topics. Please check them out.
Client Profile
We've made a number of improvements to the client profile in
.NET 4. I love the ease of switching between client profile and the
full profile should we need it. I also love the small size. There's
a ton to be said here, and this blog post says it best:
Managed Extensibility Framework
The Managed Extensibility Framework (MEF or "Glenn Block's
Project"<g>) is now rolled into the full .NET framework. Not
only is it a great way to dynamically extend your own applications,
but it's also the way the IDE is extended in VS2010.
Parallel Computing
Many personal computers and workstations have two or four cores
(that is, CPUs) that enable multiple threads to be executed
simultaneously. Computers in the near future are expected to have
significantly more cores. To take advantage of the hardware of
today and tomorrow, you can parallelize your code to distribute
work across multiple processors.
In the past, parallelization required low-level manipulation of
threads and locks. Visual Studio 2010 and the .NET Framework 4
enhance support for parallel programming by providing a new
runtime, new class library types, and new diagnostic tools. These
features simplify parallel development so that you can write
efficient, fine-grained, and scalable parallel code in a natural
idiom without having to work directly with threads or the thread
pool. The following illustration provides a high-level overview of
the parallel programming architecture in the .NET Framework 4.
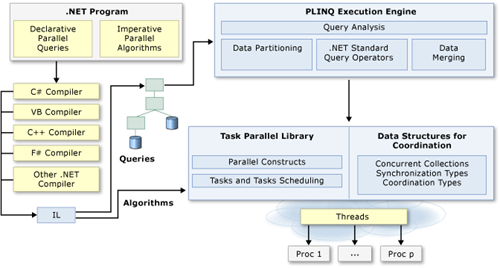
XBAP Script Access
In this day of Silverlight browser applications, you may wonder
about what's going on with Xbaps. It turns out that a good number
of customers are using them in their own applications. I talked
with several at PDC09. One thing we've done to improve the xbap
experience and to help give xbaps a bit more parity with their
Silverlight cousins is providing script access. Xbaps can now
interop with script on the browser.
For an overview of what's new in .NET 4 itself, check out the MSDN page on .NET 4.
Summary and Feedback
I'm really excited about this release of Visual Studio and .NET
4. There's tons of goodness here both in the tooling and in the
frameworks. Round it out with the pending release of Silverlight 4,
and I'm in developer heaven :)
There's more coming in WPF-land as well. Later this year we'll
see more news on the Ribbon control as well as some awesome touch
libraries from the Surface team.
We're also right in the middle of WPF v.next planning. If
there's something you want to see in the next version, please go and vote it up on the WPF uservoice
voting site.
I hope you enjoy this release as much as I have. This is my
first release as a 'softie, and I'm "super excited" to have been
here for it :)