Starting with Windows Vista, the open and save file dialog boxes
have a panel on the left side containing favorite links. These
links are known as "Custom Places". Previously, the built-in
Windows dialogs were available only if you used the Windows Forms
versions. However, starting with WPF 4 on Windows 7 and Windows
Vista support of native file dialogs with custom places via the
FileDialogCustomPlace and FileDialogCustomPlaces classes can be
found in the Microsoft.Win32 namespace right in the
PresentationFramework dll.
Using Standard Custom Places
The framework includes a number of built-in custom places,
representing the usual system and user locations on the machine,
plus the local and roaming profile locations. To use them, simply
use the static instances provided by the FileDialogCustomPlaces
class.
private void SaveButton_Click(object sender, RoutedEventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
dialog.CustomPlaces.Add(FileDialogCustomPlaces.LocalApplicationData);
dialog.CustomPlaces.Add(FileDialogCustomPlaces.Templates);
dialog.ShowDialog();
}
When displayed, the dialog will have two custom places shown at
the top left (refer to screen shot in the next section): the local
application data folder and the templates folder.
The standard places supplied are (from MSDN) :
Name |
Description |
Contacts |
Contacts folder for the current
user. |
Cookies |
Internet cookies folder for the
current user. |
Desktop |
Folder for storing files on the
desktop for the current user. |
Documents |
Documents folder for the current
user. |
Favorites |
Favorites folder for the current
user. |
LocalApplicationData |
Folder for application-specific data
that is used by the current, non-roaming user. |
Music |
Music folder for the current
user. |
Pictures |
Pictures folder for the current
user. |
ProgramFiles |
Program Files folder. |
ProgramFilesCommon |
Folder for components that are shared
across applications. |
Programs |
Folder that contains the program
groups for the current user. |
RoamingApplicationData |
Folder for application-specific data
for the current roaming user. |
SendTo |
Folder that contains the Send To menu
items for the current user. |
StartMenu |
Folder that contains the Start menu
items for the current user. |
Startup |
Folder that corresponds to the Startup
program group for the current user. |
System |
System folder. |
Templates |
Folder for document templates for the
current user. |
While the standard places will suffice for most applications,
especially since they include the local application data folder,
you may wish to provide additional locations. This is accomplished
via the FileDialogCustomPlace class.
Adding Your Own Places
Sometimes the built-in places aren't sufficient for all the
locations you'd like to surface to the user. For example, I have
some software recording/sequencing applications. Many of those
share the same folder for plug-ins, known as VSTs. I may want to
provide the ability for the user to quickly locate that folder in
the open and save file dialogs. This is accomplished by creating an
instance of the FileDialogCustomPlace class.
private void SaveButton_Click(object sender, RoutedEventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
string appSpecialPath = @"C:\Program Files (x86)\Image-Line\FL Studio 9\Plugins\VST";
FileDialogCustomPlace place = new FileDialogCustomPlace(appSpecialPath);
dialog.CustomPlaces.Add(place);
dialog.CustomPlaces.Add(FileDialogCustomPlaces.LocalApplicationData);
dialog.CustomPlaces.Add(FileDialogCustomPlaces.Templates);
dialog.ShowDialog();
}
The FileDialogCustomPlace class constructor takes in either a
string path or a GUID which refers to one of the built-in places.
In this case, I supplied it with the VST plug-in folder. When run,
the dialog looks like this:
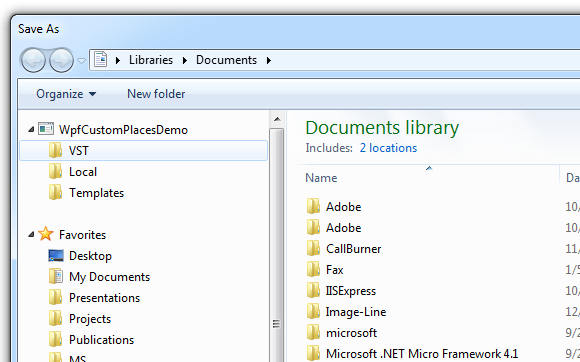
Note that the name of the folder is displayed using normal
Windows mechanisms: you get just the name of the final folder in
the path.
Also note that on Windows XP, the custom places will have no
effect. The feature is gracefully dropped.