A few weeks back, my friend Morten Neilsen tweeted
that he was able to get a .NET console app running on the Intel
Galileo with Windows. I was curious because that's not a scenario
we thought would work. So, I thought I'd try it out myself.
Please note that .NET is not currently supported on the Intel
Galileo, but it's still fun to experiment and see what works and
what doesn't.
To join the Windows on Devices program and code for the Galileo,
purchase an Intel Galileo Gen 1 from Amazon or another retailer and
sign up at http://windowsondevices.com
. From there, follow the machine setup and Galileo setup steps for
people who have their own board.
Project setup
In Visual Studio 2013, I created a new C# Console Application
project.
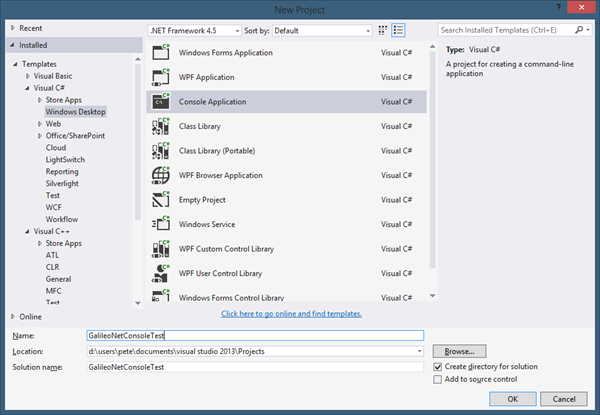
I left everything at the defaults. Once in the project, I wrote
some simple code (most of .NET is unsupported and/or not present,
so the code does need to be simple)
using System;
namespace GalileoNetConsoleTest
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("This is a .NET Test");
}
}
}
I then changed the build properties to compile only for x86.
This may be an optional step, but the Galileo is 32 bit x86
only.
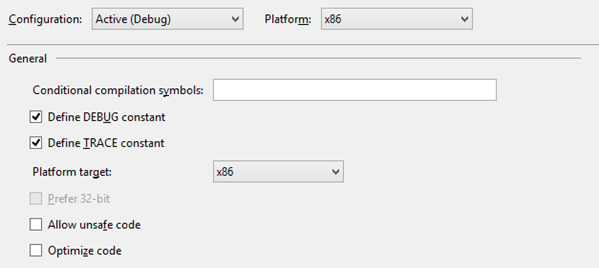
Then, I simply copied the file to my Galileo. I use a network
drive mapped to C$ ; it is Windows afterall.
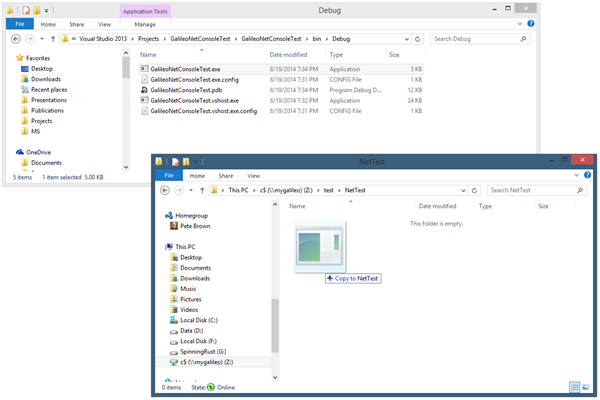
Then, in my open Telnet session, I ran the app.
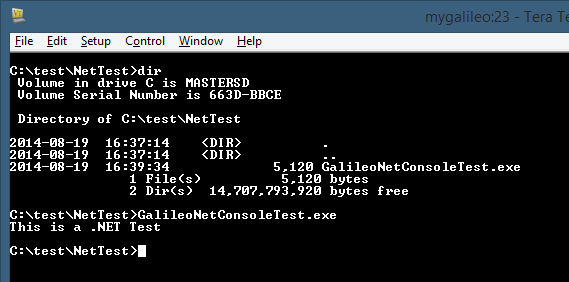
Success!
Granted, that was a super simple app, but it's nice to see that
it works.
So what works?
You saw that the basic console output works. Similarly, the core
language constructs work, as should most/all things in
mscorlib/mscoree. The instruction set for the Galileo is missing
some things that .NET generally relies upon, however, so most
non-trivial code is not expected to work. For example. if you try
to use System.Diagnostics.Debug, you'll get a
TargetInvocationException saying that System is not present. Same
thing with, say, Console.Beep().
You can do a lot of the same types of programs we used to write
back when we first learned programming, however. Here's an example
that includes a few things similar to the early programs many of us
did on the C64.
using System;
namespace GalileoNetConsoleTest
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("This is a .NET Test");
for (int i = 0; i < 50; i++)
{
Console.Write(i + ", ");
}
Console.WriteLine();
Console.Write("What is your name? ");
var name = Console.ReadLine();
Console.WriteLine();
Console.WriteLine("Hello there, " + name);
string rev = string.Empty;
for (int i = name.Length - 1; i >= 0; i-- )
{
rev += name[i];
}
Console.WriteLine("Your name reversed is " + rev.ToLower());
Console.WriteLine("It is now " + DateTime.Now.ToLongTimeString());
var rand = new Random();
var dieroll = rand.Next(5) + 1;
Console.WriteLine("You rolled " + dieroll);
}
}
}
Copied it over as before, and ran it from the Telnet session
window:
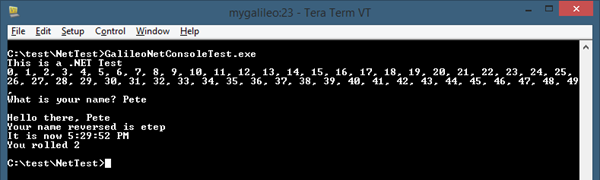
Beautiful. :)
Note that my Galileo is on Pacific time, not Eastern time. I
didn't pay much attention to that until this little sample. It's
easy to change the actual time using the Time command, but setting
the time zone requires a bit more work with reg files, or an
additional app.
I haven't gone through to figure out exactly what works and what
doesn't. However, for now, you can make the assumption that if it's
outside of System, it won't work, and if it's inside of System, it
may work, depending on which DLL it's implemented
in.
Next steps
This is all preliminary stuff. The Windows on Devices program is
in its early stages, and as such, Wiring is your best bet in terms
of the most mature part of the developer platform. That said, it's
nice to see that we can sneak a little .NET on there if we want to
:)
As mentioned
in the FAQ, our goal with the Windows on Devices program is not
just Wiring, but also the Universal App model (headless, of
course). For now, it's fun to explore to see how far we can go with
both the supported and unsupported features of the current
platform.